Using MQTT in Unity with M2MqttUnity Library: A Step-by-Step Guide
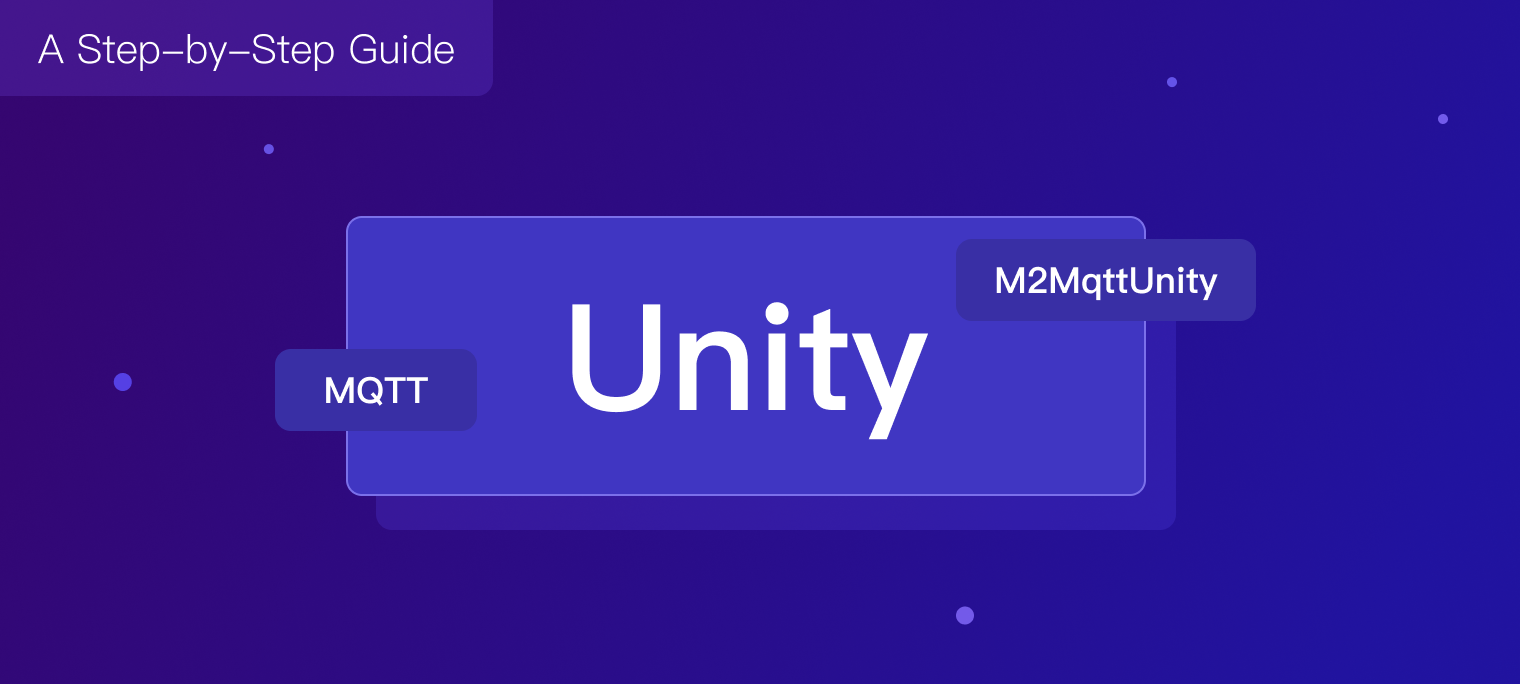
Table of Contents
Unity is a popular game engine that is used to create a wide range of games and simulations. MQTT is a lightweight messaging protocol based on publish/subscribe model, specifically designed for IoT applications in low bandwidth and unstable network environments.
MQTT can be used in Unity for a variety of purposes, including AR (Augmented Reality) games, IoT-enabled games that utilize sensors and other devices, and remote control of games and simulations. For example, in AR games, MQTT can be used to receive information about the world from the user's device, such as the locations of planar surfaces, the detection of objects, people, faces, and so on.
This blog provides a simple Unity3d project for using M2MQTT with Unity. The project includes an example scene with a user interface for managing the connection to the broker and testing messaging.
Prerequisites
Install Unity
Download the installers for all major platforms from the Unity website: https://unity.com/download.
Prepare an MQTT Broker
Before proceeding, please ensure you have an MQTT broker to communicate and test with.
In this blog post, we will use the free public MQTT broker at broker.emqx.io
.
Server: broker.emqx.io
TCP Port: 1883
WebSocket Port: 8083
SSL/TLS Port: 8883
Secure WebSocket Port: 8084
The free public MQTT broker is exclusively available for those who wish to learn and test the MQTT protocol. It is important to avoid using it in production environments as it may pose security risks and downtime concerns.
M2MQTT for Unity
M2MQTT library is a simple Unity3d project for using M2MQTT with Unity. It includes an example scene with a UI for controlling the connection to the broker and for testing messaging. In this blog post, we will use the example scene to illustrate how to use MQTT to create an application that communicates with an MQTT broker.
To begin, download the repository from GitHub.
Git clone https://github.com/CE-SDV-Unity/M2MqttUnity.git
Import the Example Scenes
Next, create a new Unity project in UnityHub.
Then, copy the M2Mqtt
and M2MqttUnity
folders from the downloaded M2MQTT repository to the Assets
folder of the new Unity project.
The library provides a test scene called M2MqttUnity_Test
, located in the M2MqttUnity/Examples/Scenes
folder. By inspecting the scene, we can see that the only script used to setup the MQTT Client is M2MqttUnityTest.cs
, which is attached to the M2MQTT GameObject in the scene. However, this script is linked with other classes of the main folder M2Mqtt
.
Connect and Subscribe
Press Play
to run the application. In the Game
tab, you will see a default broker which is already offline in the Broker Address input box. You should replace it with the public MQTT broker (broker.emqx.io
) we have prepared beforehand.
After replacing the address, click on "Connect" to establish a connection to the public MQTT broker.
The corresponding code is very simple. It creates a connection to broker.emqx.io
, and then subscribes to the topic "M2MQTT_Unity/test".
public void SetBrokerAddress(string brokerAddress)
{
if (addressInputField && !updateUI)
{
this.brokerAddress = brokerAddress;
}
}
public void SetBrokerPort(string brokerPort)
{
if (portInputField && !updateUI)
{
int.TryParse(brokerPort, out this.brokerPort);
}
}
public void SetEncrypted(bool isEncrypted)
{
this.isEncrypted = isEncrypted;
}
public void SetUiMessage(string msg)
{
if (consoleInputField != null)
{
consoleInputField.text = msg;
updateUI = true;
}
}
public void AddUiMessage(string msg)
{
if (consoleInputField != null)
{
consoleInputField.text += msg + "\\n";
updateUI = true;
}
}
protected override void OnConnecting()
{
base.OnConnecting();
SetUiMessage("Connecting to broker on " + brokerAddress + ":" + brokerPort.ToString() + "...\\n");
}
protected override void OnConnected()
{
base.OnConnected();
SetUiMessage("Connected to broker on " + brokerAddress + "\\n");
if (autoTest)
{
TestPublish();
}
}
protected override void SubscribeTopics()
{
client.Subscribe(new string[] { "M2MQTT_Unity/test" }, new byte[] { MqttMsgBase.QOS_LEVEL_EXACTLY_ONCE });
}
Publish MQTT Messages
We will use MQTT Client Tool - MQTTX as another client to test the message publishing and receiving.
After connecting, click on Test Publish
. You will see that the "Test message" has been received by both the game client and MQTTX.
The corresponding code is to use publish() function to publish a message “Test message” to topic "M2MQTT_Unity/test".
public void TestPublish()
{
client.Publish("M2MQTT_Unity/test", System.Text.Encoding.UTF8.GetBytes("Test message"), MqttMsgBase.QOS_LEVEL_EXACTLY_ONCE, false);
Debug.Log("Test message published");
AddUiMessage("Test message published.");
}
Receive Messages
When using MQTTX to send another test message, the client will receive it and we can see it in the Game tab.
The corresponding code is to decode, process and display the message on the Game tab.
protected override void DecodeMessage(string topic, byte[] message)
{
string msg = System.Text.Encoding.UTF8.GetString(message);
Debug.Log("Received: " + msg);
StoreMessage(msg);
if (topic == "M2MQTT_Unity/test")
{
if (autoTest)
{
autoTest = false;
Disconnect();
}
}
}
private void StoreMessage(string eventMsg)
{
eventMessages.Add(eventMsg);
}
private void ProcessMessage(string msg)
{
AddUiMessage("Received: " + msg);
}
protected override void Update()
{
base.Update(); // call ProcessMqttEvents()
if (eventMessages.Count > 0)
{
foreach (string msg in eventMessages)
{
ProcessMessage(msg);
}
eventMessages.Clear();
}
if (updateUI)
{
UpdateUI();
}
}
Summary
This blog post provides a guide to creating an application that communicates with an MQTT broker using Unity. By following this guide, you will learn how to establish a connection, subscribe to topics, publish messages, and receive real-time messages using the M2MQTT library.
Join the EMQ Community
To dive deeper into MQTT, explore our GitHub repository for the source code, join our Discord for discussions, and watch our YouTube tutorials for hands-on learning. We value your feedback and contributions, so feel free to get involved and be a part of our thriving community. Stay connected and keep learning!